Rust Multithreading
Introduction
I've always wanted to look at multithreading more in depth, especially in Rust. I decided to do it with a recent project I took on: building a port sniffer. This project not only highlighted Rust's efficiency in handling concurrency but also its inherent safety features that make it a standout choice for such tasks. Just as a brief description. A port sniffer checks which ports on a particular device are open and are sending/receiving data. Think of it as your PC being a house and this program being a spy which checks which doors/windows are open. Obviously, you might think it’s unethical, but consider it more as a homeowner checking for any unlocked doors – it's about security, not nosiness!
Rust's Safety Guarantees
What sets Rust apart in concurrent programming is its rigid stance on safety. The language's ownership model brilliantly resolves common multithreading dilemmas, like data races, at compile time, ensuring a level of safety that's hard to achieve in many other languages.
Building a Port Sniffer in Rust
In my port sniffer project, I utilized Rust's concurrency features to scan multiple ports simultaneously. Here's a snippet of the code:
// ... scanning ports ...
async fn scan(tx: Sender<u16>, port: u16, ipaddr: IpAddr){
match TcpStream::connect((ipaddr, port)).await{
Ok(_) => {
print!(".");
io::stdout().flush().unwrap();
tx.send(port).unwrap();
}
Err(_) => {}
}
}
#[tokio::main]
async fn main() {
// ... [Code for initializing and running the port sniffer] ...
}
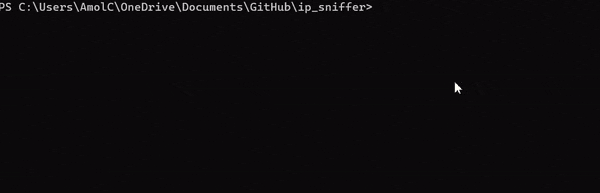
The output contains all the ports my PC is using.
This project involved using the tokio crate for asynchronous programming and bpaf for command-line argument parsing. The real challenge and learning came from managing multiple asynchronous tasks to scan a range of ports efficiently and safely. Rust's powerful yet straightforward concurrency model made it possible to implement this complex functionality with relative ease.
Rust's Approach to Multithreading
Rust's multithreading isn't just about launching threads; it’s about a thoughtful design that guarantees safety without compromising performance. For instance, in the port sniffer project, managing shared state and ensuring thread safety were crucial. Rust's type system and ownership rules played a vital role in avoiding common pitfalls like shared mutable state issues.
Conclusion
Working on the port sniffer project and learning more about multithreading was both challenging and rewarding. It reinforced the importance of understanding the intricacies of concurrency and the value of a language that navigates these complex waters safely. It's something that has not only honed my programming skills but also deepened my understanding for concurrent programming.